Self-Documenting Code
READ WHOLE ARTICLE
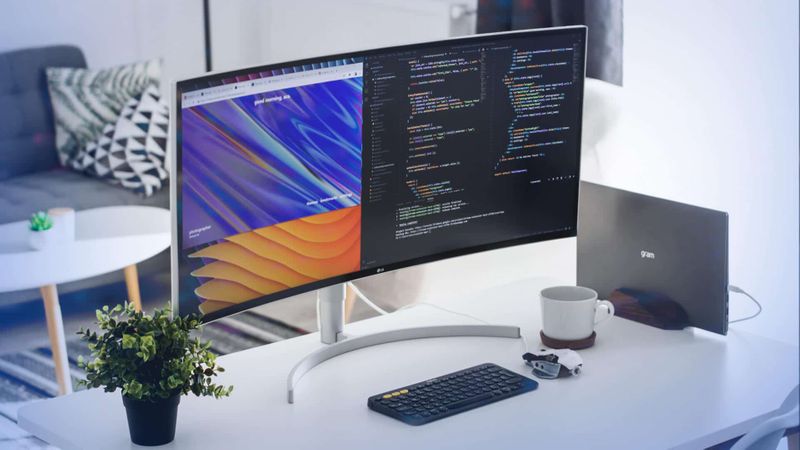
A complex and practical program requires great skill. Practice shows that reading and understanding computer programs is much more difficult than writing them. For each project it is important to have documentation. The only document that describes your code completely and correctly is the code itself. It doesn’t mean that better description is impossible, but more often it is the only documentation that is at your disposal.
Therefore, you should make every effort to make this documentation good — such which everyone can read. The fact is that the author of the code is not the only person who needs to understand it. Programming languages serve as a means of communication for us. Communication should be clear. Due to the clarity of the code, its quality grows as errors become less likely (they are more noticeable),and maintaining the code is cheaper – less time is required to study it.
Self-documenting code is easy to read. It is understandable without additional documentation. To facilitate the understanding of the code can be done in many ways. Some techniques are elementary and are included in the basics of training programmers, others are more sophisticated, and possession comes with experience. So what is self-documenting code? Let’s find out!
What is self-documenting code?
In theory, the code of a good engineer should be so clear and readable that he simply does not need any documentation. Frankly speaking, it’s utopia. For each project it is important to have documentation. In programming, self-documenting coding source code and UIs pursue naming conventions and organized programming conventions that empower utilization of the framework without earlier explicit knowledge. Speaking about web development, it refers to a site that exposes the whole procedure of its creation through open documentation, and whose open documentation is a piece of the development procedure.
Self-documenting code can improve the readability of your codebase. It basically means that you don’t have to write comments to explain what your code is doing.
Pros and cons of self-documenting code
So what can we win or lose by using self-documenting code in our development procedure? The benefits are quite obvious:
- your codebase is easy to understand;
- improved readability of your code;
- make developers think of the only responsibility;
- a developer doesn’t have to think about leaving comments all the time.
However, self-documenting code doesn’t fit to every project and built. It’s not so all-purpose. Here are the reasons:
- programming is full of stuff that couldn’t be self-documented. There is a big difference between what you see and read, and documentation;
- the more complicated your app is, the less self-documenting code will be understandable.
Considering all the information above, many engineers believe the comments more than self-documenting code.
Self-documenting code vs Comment?
Usually people who argue on this question can be divided into 2 groups:
- those who think that developers should always comment the code and update or sync their notes;
- those who are fed up with not regularly updated and sync code, so struggle to make comments useless by self-documenting code.
Comment side thinks that self-documenting one just doesn’t bother of code at all and laid-back when their opponents don’t believe comments because of their twisting truth.
Let’s compare them to end this old war:
Category | Self-documenting code | Comments |
Easy to do | NO Only an experienced developer can write high quality self-documenting code. | YES BUT a dev should remember about it. |
Descriptiveness | Clear but without details. | Depends on an author. |
Time limits | Not time-consuming. A developer writes coding and documenting it simultaneously. He/She needs to be careful about structure. | Time-consuming. A developer should comment every line or part after writing it, so spend more time. |
Any code must be self-documented. In a good self-documented code, you don’t need to explain each line, because each identifier (variable, method, class) has a clear semantic name. Having more comments than necessary, in fact, it makes code difficult (!) to read, so if a developer records:
- documentation comments (Doxygen, JavaDoc, XML comments, etc.) for each class, member, type, and AND method comments;
- comments clearly on any parts of the code that are not self-documenting;
- writes a comment for each block of code that explains the intent, or what the code does at a higher level of abstraction
its code and documentation are fine and clear. Note that presence of self-documenting code does not mean that there should be no comments, but only that there should be no unnecessary comments. The fact is that, reading the code (including comments and comments to the documentation), you should immediately understand what the code does and why. If the self-documenting code takes longer than the commented code, it is not self-documented.
10 practices for writing self-documenting code
We can divide all the techniques of writing self-documenting code into three broad categories:
- structural, in which the structure of the code or directories is used to clarify its purpose;
- related to naming, such as naming functions and variables;
- syntax related in which individual language features are used for this purpose.
Now we will show structural, naming and syntax practices for writing self-documenting code in JavaScript.
Structural practices
Structural category is the first we are going to look at. For better clarity, code is shifted around which is the main structural change.
1. Code is moved into a function
Existing code is moved to a new function which means that we extract the code into the new function. Let’s guess the meaning of the following line:
var width = (value - 0.5) * 16;
We can guess but it’s not so clear, it’s more useful to put a comment here. BUT we can move the code into a new function:
var width = emToPixels(value);
function emToPixels(ems) {
return (ems - 0.5) * 16;
}
Now the function’s name describes its purpose so the code doesn’t need clarification anymore. We made the code self-documenting.
2. Expression is replaced with a variable
This practice is almost the same as the previous one but we use a variable instead of the function. Let’s take a look:
var isVisible = el.offsetWidth && el.offsetHeight;
if(!isVisible) {
}
If the logic is really specific to a definite algorithm, this practice is better than extracting a function.
Mostly developers use mathematical expressions in this technique:
return a * b + (c / d);
This line can be clarified with splitting it:
var multiplier = a * b;
var divisor = c / d;
return multiplier + divisor;
Complex expressions are moved into variables so the algorithm becomes more understandable.
3. Class and module interfaces
Class and module interfaces can serve as a documentation. For instance:
class Box {
setState(state) {
this.state = state;
}
getState() {
return this.state;
}
}
Here it’s clear what the functions do because of their names but it’s not clear how to use them.
Let’s change them a bit:
class Box {
open() {
this.state = 'open';
}
close() {
this.state = 'closed';
}
isOpen() {
return this.state === 'open';
}
}
The internal representations is still with the same property and we just changed the public interface. Now we see the usage properly.
4. Group your code
You can gather different clues of code to document it in JavaScript of any other language. For instance, put variables closer to the place where they would be used and group their uses together. It helps to understand a relationship between various clues of the code.
Let’s see the example:
var foo = 1;
blah()
xyz();
bar(foo);
baz(1337);
quux(foo);
In the second example here, we can easily understand the dependence of different parts of the code thanks to foo uses grouped together.
Naming practices
Let’s move to using naming things to change our code to self-documenting.
5. Give another name to the function
To make your functions’ names more understandable, we prepared a few rules to follow:
- Omit to use obscure words:
handleButtons(), manageLinks()
- Use active verbs like ”send”:
cutLawn(), sendFolder()
6. Give other names to variables
To make a code line clear, rename variables and don’t use shortcuts, e.g. a if it’s not about counters. Also you should include the expected units if there are any numeric parameters. For instance, widthPx instead of width to indicate the value in pixels.
7. Try to follow the same naming conventions
For instance, if there is a specific type of object, give it the same name:
var element = getElement();
Don’t change the name, your self-documenting code will become confusing:
var node = getElement();
Syntax practices
Such practices could be more language specific. For instance, Ruby enables doing all syntax things which should be omitted, in general. We’ll show a few ones for JavaScript.
8. Avoid utilizing syntax tricks
Such trick really confuse everybody. Check it out:
imStrange && doMagic();
It’s the same as this option but more clear:
if(imTricky) {
doMagic();
}
9. Prefer to use named constants
Choose a constant to use, if you have some special values(numbers or string values) in the code.
const BUY_HAPPINESS = 42;
10. Omit boolean flags
Code can become vague because of boolean flags, e.g.:
myStuff.setData({ x: 1 }, true);
Try to guess the myStuff meaning. Is it hard? Yes, so that you should add another function or rename it:
myStuff.mergeData({ x: 1 });
Final Words
Self-documenting code is not a myth but a must-write in any type of IT development area. It’s no use considering that your code won’t be touched or redone one more time. Moreover, writing self-documenting code helps developers not only to understand it easily but to find code errors. It doesn’t fit all the types of development: the more complex a build is, the more you need to add comments to self-documenting code. That’s the best option to rely on. For every new project we take from the business idea, Multi-Programming Solutions sharps all the code lines to be easy-to-read and comprehend. We prefer self-documenting code and comments unconditionally because our developers know its value and necessity. We think that these 10 practices are worth using if you decided to be more single responsive and make your code self-documenting!
36 Kings Road
CM1 4HP Chelmsford
England