PWA for Shopify Stores Using Angular
READ WHOLE ARTICLE
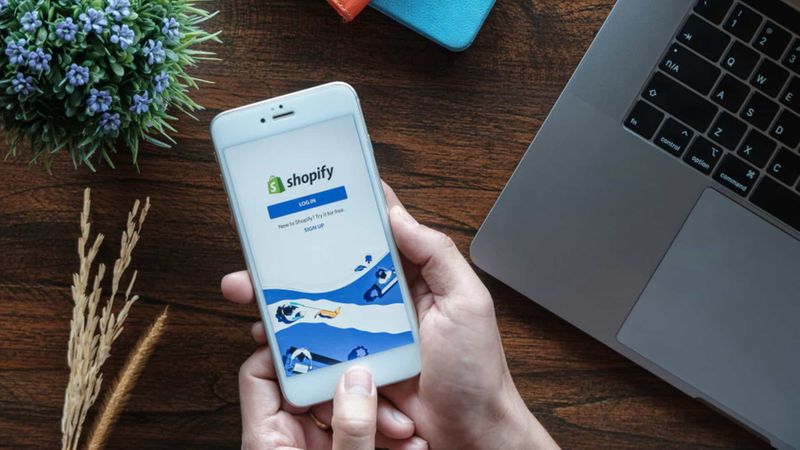
Progressive Web Apps (PWA) is a popular software concept introduced in 2015 by Google that brought regular web apps on desktop and mobile to a whole new level. Employing Service Workers, Web App manifest, push-notifications, HTTPS, App Shell, etc., Google’s PWA policies imply these advanced solutions to strictly fit the following standards:
- Reliable (parts of functionality are available offline);
- Fast (in PWAs, most part of interactive elements and dynamic content is refreshed on the client-side);
- Engaging (a PWA provides smooth and inviting user experience).
You can create a profitable, user-pleasing PWA solution for your Shopify-based eCommerce store as well. We’ll tell you how, but first, let’s figure out some essentials.
How Does a PWA Work?
Progressive web applications combine the best features of native and web software – the best of both worlds, if you may. At the same time, they are developed with the combination of regular means, such as HTML and JavaScript, as well as tools specific to PWAs – the mentioned Service Worker, Push Notifications, HTTPS, App Shell, etc.
If we go a bit deeper into the underlying mechanisms of PWA performance, everything is as follows.
When a user launches a site, Service Worker “registers” it, while monitoring all the clicks on the pages that the user carries out. With each click, a new instance of Service Worker is created, during which the installation event is triggered and all possible server requests are answered. After that, everything covered by the App Shell is cached. Generally, Service Worker monitors all user actions on the site, filling in the View data during the dynamic update of content.
As such, event handlers are started automatically, and Service Worker switches to standby mode after each handler launch (until the next event is triggered). This preload provides an instant response to user actions within the PWA, even if there is no network connection at the moment.
Learn even more details on the work principles of PWA in another dedicated feature.
Advantages of Using Progressive Web Apps for Shopify Stores
- Accessible, accelerated development. According to the specialized developers, Progressive Web Apps are quite simple to develop, despite the impressive stack of technologies involved (including, again, App Shell, Push Notifications, etc.). Native software is still much more difficult to create because developers need to know the specifics particular mobile platforms instead of simply going with the usual JS, CSS and HTML. For the same reason, in the case of PWA, developers no longer need to create a separate code base;
- Simpler promotion & extended TA reach. PWAs are more accessible overall, which widens your usual target audience reach without increasing promotion costs. Progressive Web Apps are also better indexed by search engines: in addition to high performance, Google also pays attention to a security protocol – all advanced web applications use HTTPS by default. We can say that PWAs “promote themselves”: in order to demonstrate something from your Shopify store, a user just needs to share the link (you do not need to install the application).
How to develop your own Shopify apps
- All-around compatibility. Thanks to the cross-platform technology, you can develop one solution that will work on any device and support any platform, via any preferred browser;
- Maximum interactivity & speed of user response. As we mentioned above, PWAs work with a low-speed or no network connection at all. App Shell allows for ridiculously fast content loading speeds. And offline access boosts conversion rates in the long run because even if there is no access to the network, a user will still be able to add goods to the cart or implement other target actions;
- Engaging user experience. PWAs provide an unrivaled level of user experience, which means that visitors to your store will most likely want to return to it again. For example, users can add bookmarks and save their favorite PWAs on the home screen without having to install the app (and according to statistics, every click necessary to perform a target action lowers your profits by 20%). PWA don’t need to be either installed or updated – you only use the solution, all the other stuff happens on the other side of the screen;
- Tech advancement. PWAs are based on the most up-to-date technology stack: push notifications, advanced content caching, background content updates, and much more, which means users get the cream of what the modern web development industry has to offer. There are also hardware accelerated 2D/3D graphics (achieved through WebGL or HTML5 Canvas), animation at 60 frames per second, launch in fullscreen mode, access to the buffer, access to the file system and reading of user-selected files in any browser;
- Increased user security. PWAs are considered highly secure web solutions, in big part, due to the HTTPS reinforcement;
- Cost-efficient development. PWA development costs much lower than the development of native software, which means that this format is the best solution for those customers who do not have the opportunity to order the development of a full-fledged application.
Yes, the PWA format may not be a full-on replacement for regular mobile software, as progressive apps are unable to reach for hardware functionality of smart devices.
However, a PWA solution integrated with your Shopify store theme will serve as a great additional effort in boosting conversion rates and positioning your eCommerce in the market more prominently.
By the way, the top platforms for transforming your existing eCommerce into a Progressive Web App for Shopify include solutions by Shop Sheriff, AmpifyMe, and Litefy.
A Detailed Tutorial on How to Create a PWA for Shopify Using Angular
Make sure your website fits PWS policies
Before you get to the implementation of a PWA for Shopify based on Angular, you need to make sure that the existing resource corresponds with some standard requirements and has:
- SSL certificate;
- mobile version or adaptive design;
- Service Worker in the software code;
- separate URLs for separate pages.
Connect an Angular UI design solution
In order to connect Angular Material, use these commands:
$cd NgPwa
$add @angular/material
Connect & configure HTTPClient
HTTPClient is an Angular service used for the creation of new scripts for processing HTTP requests. For starters, connect it like so:
/*...*/
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
/*...*/
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Next, you need to connect CORS and deactivate remote data source reading (we do this below with the help of Jargon GitHub Simplified JavaScript). To do all that, you need to open the file at src/app/api.service.ts and specify the following command there:
$ng g service api
Then, define dependencies with Observable class:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
export interface Item {
name: string;
description: string;
url: string;
html: string;
markdown: string;
}
@Injectable({
providedIn: 'root'
})
export class ApiService {
private dataURL: string = "https://www.techiediaries.com/api/data.json";
constructor(private httpClient: HttpClient) {}
get(): Observable<Item[]>{
return this.httpClient.get(this.dataURL) as Observable<Item[]>;
}
}
Now, connect Api.Service to your app. For this, simply open the file at src/app/app.component.ts and add the following there:
import { Component, OnInit } from '@angular/core';
import { ApiService } from './api.service';
import { Item } from './api.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
title = 'NgPwa';
items: Array<Item>;
constructor(private apiService: ApiService){
}
ngOnInit(){
this.getData();
}
getData(){
this.apiService.get().subscribe((data: Array<Item>)=>{
console.log(data);
this.items = data;
}, (err)=>{ console.log(err);
});
}
}
Work on the interface
Now, you can work on the basic Angular Material component – a carcass of a particular web page. For this, add the existing code in the file at src/app/app.module.ts with the following lines:
/*...*/
import {MatButtonModule} from '@angular/material/button';
import {MatExpansionModule} from '@angular/material/expansion';
import {MatToolbarModule} from '@angular/material/toolbar';
@NgModule({
declarations: [
AppComponent
],
imports: [
/*...*/
MatButtonModule,
MatExpansionModule,
MatToolbarModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Next, open src/application/app.component.html and replace its contents with the following:
<mat-toolbar color="primary">
<mat-toolbar-row>{{title}}</mat-toolbar-row>
</mat-toolbar>
<mat-accordion>
<mat-expansion-panel *ngFor="let item of items">
<mat-expansion-panel-header>
<mat-panel-title>
{{item.name}}
</mat-panel-title>
</mat-expansion-panel-header>
<div [innerHTML]="item.html"></div>
<a mat-button [href]="item.url">Go to full article</a>
</mat-expansion-panel>
</mat-accordion>
Connect a PWA-compliant stack of technologies
It is noteworthy that none of the PWA-specific technologies is contained in Angular by default (we are talking about Service Worker, Push Notifications, Web App manifest, HTTPS, App Shell, etc.). You will have to connect each manually.
How to create PWA using Angular
Summary
Summarizing all that up, as you can see, the PWA Angular format brings a lot of advantages to Shopify eCommerce owners, including excellent UX, high conversion rates, and boosted SEO. You can also turn your Shopify store into a Progressive Web App to extend your business capacities – contact us and we’ll gladly do implement your project.
36 Kings Road
CM1 4HP Chelmsford
England